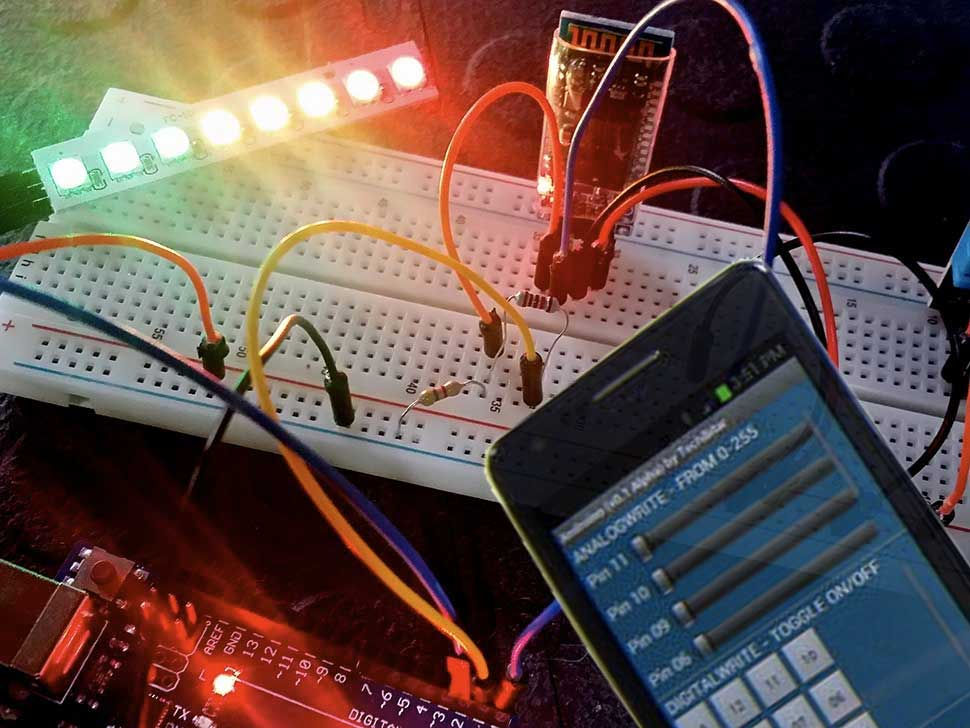
OVERVIEW
We already saw how to use IR and RF remote controls in past tutorials, this time we will see how to use Bluetooth and an Android device to control your next Arduino project.
We will be using the popular HC-06 bluetooth module, this module makes it possible to send commands and retrieve information from the UNO.
There’s also the HC-05 version of this module that is available.
The main difference between the two is that the HC-05 can act as Master as well as Slave.
The HC-06 is Slave only.
In most situations the HC-06 will do the job.
For example you would need to use the HC-05 is if you need the Arduino to actually control something on it’s own.
In this tutorial we will retrieve the temperature and humidity information from the sensor connected on the UNO and display it on the free Android app that we will be using called ‘Ardudroid’.
We will also control a WS2812 RGB stick using a PWM slider from the Android device.
PARTS USED
DHT-11 Temperature sensor
Arduino UNO
HC-06 Bluetooth Module
These are Amazon affiliate links...
They don't cost you anything and it helps me keep the lights on
if you buy something on Amazon. Thank you!
CONNECTIONS
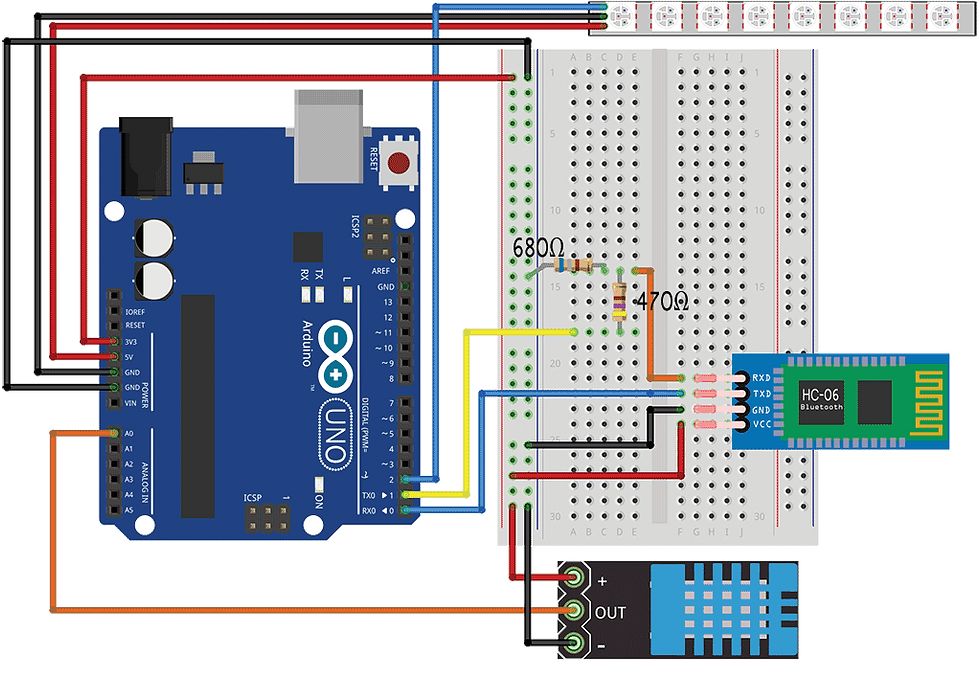
There are many different ways to connect these HC-0x Arduino modules.
Some involves using libraries to communicate with the modules using Software Serial connections to enable you to still program (send sketches) to the UNO without having to unplug the Bluetooth module.
The tradeoff is that if you use these methods you lose some Pins on the UNO which are then used to connect the module.
Instead, we will just disconnect the wire on Pin 0 of the UNO when we want to program it.
This way we can connect the module to the default Pin 0(RX) and Pin1(TX) and still have all the other pins available to play with.
As you can see in the schematic above, we are using some resistor (470Ω and 680Ω) to drop the voltage of the UNO TX pin from 5V to around 3V.
This is important since the HC-06 uses 3.3V logic and using the default 5V of the UNO would damage the HC-06 module.
Also we power the module using the 3.3V from the UNO not 5V, even if the HC-06 supports up to 6V.
From our testing we noticed that if powered from 5V the ‘Get Data’ window on the ArduDroid app would not work properly.
THE CODE
Our sketch is based on the ArduDroid skeleton sketch available here, we modified it to include the functions we wanted to use in this tutorial.
We will add the code to light up the WS2812 RGB stick connected to Pin 2 by mapping the PWM readings from the #6 Slider from ArduDroid.
Also write some code to get the temp and humidity readings from our DHT-11 module and display them in the ‘Get Data’ window on the Android device.
*Please note that these are just quick examples of what you could do using Bluetooth, you can pretty have your UNO do anything you want when you send a command using the ArduDroid app.
As always please have a look at our Tutorial Video for more information.
/* Start of Code */
#include "dht.h" // DHT11 library
#include "FastLED.h" // FastLED library
#define NUM_LEDS 8 // # of WS2812 LEDs
#define DATA_PIN 2 // WS2812 DIN pin
#define dht_apin A0 // DHT11 pin
//ArduDroid variables
#define START_CMD_CHAR '*'
#define END_CMD_CHAR '#'
#define DIV_CMD_CHAR '|'
#define CMD_DIGITALWRITE 10
#define CMD_ANALOGWRITE 11
#define CMD_TEXT 12
#define CMD_READ_ARDUDROID 13
#define MAX_COMMAND 20 // max command number code. used for error checking.
#define MIN_COMMAND 10 // minimum command number code. used for error checking.
#define IN_STRING_LENGHT 40
#define MAX_ANALOGWRITE 255
#define PIN_HIGH 3
#define PIN_LOW 2
dht DHT; //DHT11 Init
CRGB leds[NUM_LEDS]; // FastLED Init
String inText; // used to send txt command from Android
void setup() {
FastLED.addLeds<NEOPIXEL,DATA_PIN>(leds, NUM_LEDS); //Setup FastLED
FastLED.clear();
Serial.begin(9600);
Serial.println("HC-06 Connected!");
Serial.flush();
}
void loop()
{
Serial.flush();
int ard_command = 0;
int pin_num = 0;
int pin_value = 0;
char get_char = ' '; //read serial
// wait for incoming data
if (Serial.available() < 1) return; // if serial empty, return to loop().
// parse incoming command start flag
get_char = Serial.read();
if (get_char != START_CMD_CHAR) return; // if no command start flag, return to loop().
// parse incoming command type
ard_command = Serial.parseInt(); // read the command
// parse incoming pin# and value
pin_num = Serial.parseInt(); // read the pin
pin_value = Serial.parseInt(); // read the value
// GET TEXT COMMAND FROM ARDUDROID
if (ard_command == CMD_TEXT){
inText =""; //clears variable for new input
while (Serial.available()) {
char c = Serial.read(); //gets one byte from serial buffer
delay(5);
if (c == END_CMD_CHAR) { // if we the complete string has been read
// add your code here
break;
}
else {
if (c != DIV_CMD_CHAR) {
inText += c;
delay(5);
}
}
}
}
// GET digitalWrite DATA FROM ARDUDROID
if (ard_command == CMD_DIGITALWRITE){
if (pin_value == PIN_LOW) pin_value = LOW;
else if (pin_value == PIN_HIGH) pin_value = HIGH;
else return; // error in pin value. return.
set_digitalwrite( pin_num, pin_value);
return; // return from start of loop()
}
// GET analogWrite (Sliders) DATA FROM ARDUDROID
if (ard_command == CMD_ANALOGWRITE) {
if (pin_num == 6) { // If #6 slider is used
int numLedsToLight = map(pin_value, 0, 255, 0, NUM_LEDS);
FastLED.clear();
for(int led = 0; led < numLedsToLight; led++) {
if(led < 3)leds[led] = CRGB::Green;
if(led >=3 & led < 6)leds[led] = CRGB::Orange;
if(led >=6)leds[led] = CRGB::Red;
}
FastLED.setBrightness(50);
FastLED.show();
}
return; // Done. return to loop();
}
// SEND DATA TO ARDUDROID (Get Data button)
if (ard_command == CMD_READ_ARDUDROID) {
// Get and send Temp & Humidity to Android
DHT.read11(dht_apin);
delay(1000);
Serial.print("T: ");
Serial.print(DHT.temperature, 0);
Serial.print("c H: ");
Serial.print(DHT.humidity, 0);
Serial.print("%");
return; // Done. return to loop();
}
}
// Select the requested pin# for DigitalWrite action
void set_digitalwrite(int pin_num, int pin_value)
{
switch (pin_num) {
case 13:
pinMode(13, OUTPUT);
digitalWrite(13, pin_value);
// add your code here
break;
case 12:
pinMode(12, OUTPUT);
digitalWrite(12, pin_value);
// add your code here
break;
case 11:
pinMode(11, OUTPUT);
digitalWrite(11, pin_value);
// add your code here
break;
case 10:
pinMode(10, OUTPUT);
digitalWrite(10, pin_value);
// add your code here
break;
case 9:
pinMode(9, OUTPUT);
digitalWrite(9, pin_value);
// add your code here
break;
case 8:
pinMode(8, OUTPUT);
digitalWrite(8, pin_value);
// add your code here
break;
case 7:
pinMode(7, OUTPUT);
digitalWrite(7, pin_value);
// add your code here
break;
case 6:
pinMode(6, OUTPUT);
digitalWrite(6, pin_value);
// add your code here
break;
case 5:
pinMode(5, OUTPUT);
digitalWrite(5, pin_value);
// add your code here
break;
case 4:
pinMode(4, OUTPUT);
digitalWrite(4, pin_value);
// add your code here
break;
case 3:
pinMode(3, OUTPUT);
digitalWrite(3, pin_value);
// add your code here
break;
case 2:
pinMode(2, OUTPUT);
digitalWrite(2, pin_value);
// add your code here
break;
// default:
// if nothing else matches, do the default
// default is optional
}
}
/* End of Code */
TUTORIAL VIDEO
DOWNLOAD
Copy the above Sketch code in your Arduino IDE software to program your Arduino.
You can download the ArduiDroid App on Google Play here.
Comentarios