How to connect and use a Nokia 5110 LCD with an Arduino
- Brainy-Bits
- Oct 23, 2020
- 3 min read
Updated: Oct 28, 2020
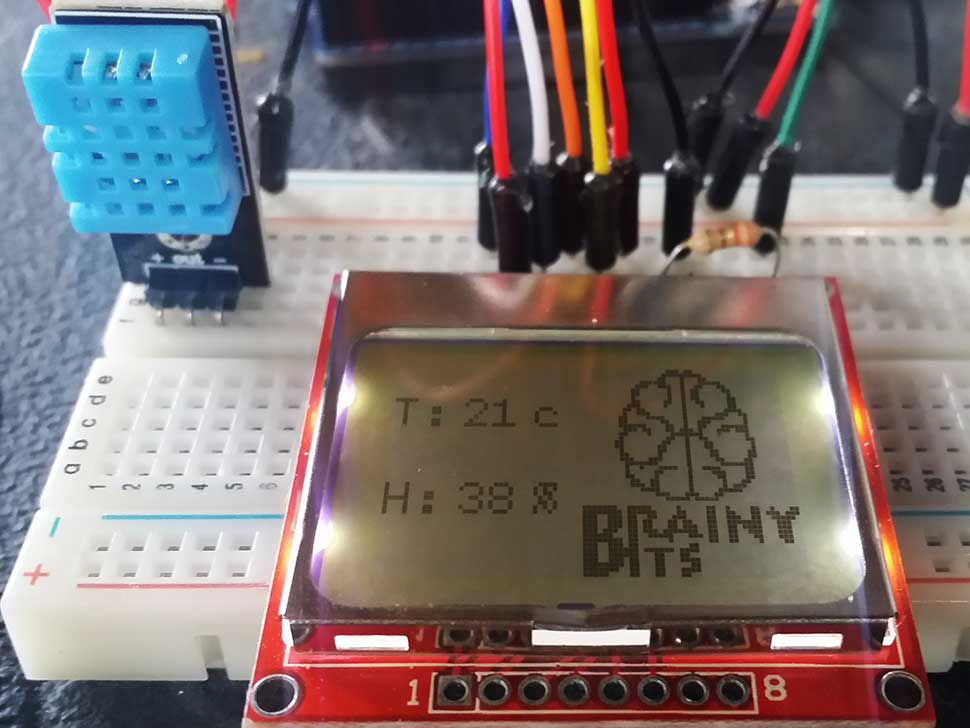
OVERVIEW
There are many tutorials out there that show how to connect and use a Nokia 5110 LCD display with an Arduino.
In this tutorial we will look at an easy way to control the 5110 by using the u8glib library.
If you want to display text / graphics and have more control of the 5110, the u8glib is great, but it does takes more memory, and is a little more complicated to use, but if you want to have more options, using the u8glib library is the way to go.
PARTS USED
DHT-11 Temperature sensor
Arduino UNO
Nokia 5110 Module
These are Amazon affiliate links...
They don't cost you anything and it helps me keep the lights on
if you buy something on Amazon. Thank you!
CONNECTIONS

The Nokia 5110 LCD uses 3.3V so we need to make sure that we connect it too the 3.3v on the Arduino not 5V.
A lot of tutorials out there say to not use the 5V logic voltage with these displays, as it may shorten the display’s lifespan.
This might be true with older versions of those displays, but in our experience we never had any problem using the digital pins of the Arduino as-is.
As you can see in the schematic above, the pins of the UNO are directly connected to the display making it very easy to connect.
THE CODE
The sketch is using the u8glib library to display the graphic and the DHT11 sensors information.
As always, please check out the tutorial video to have more information.
#include "U8glib.h"
#include "dht.h"
#define dht_apin A1
#define backlight_pin 11
dht DHT;
U8GLIB_PCD8544 u8g(8, 4, 7, 5, 6); // CLK=8, DIN=4, CE=7, DC=5, RST=6
const uint8_t brainy_bitmap[] PROGMEM = {
0x00, 0x00, 0x03, 0xB0, 0x00, 0x00, 0x00, 0x00, 0x07, 0xFC, 0x00, 0x00, 0x00, 0x00, 0x0C, 0x46,
0x00, 0x00, 0x00, 0x00, 0xFC, 0x47, 0xC0, 0x00, 0x00, 0x01, 0xCE, 0x4C, 0x60, 0x00, 0x00, 0x03,
0x02, 0x58, 0x30, 0x00, 0x00, 0x03, 0x02, 0x58, 0x10, 0x00, 0x00, 0x02, 0x02, 0x58, 0x18, 0x00,
0x00, 0x03, 0x06, 0x4C, 0x18, 0x00, 0x00, 0x07, 0x04, 0x44, 0x18, 0x00, 0x00, 0x0D, 0x80, 0x40,
0x3C, 0x00, 0x00, 0x09, 0xC0, 0x40, 0xE6, 0x00, 0x00, 0x18, 0x78, 0x47, 0xC2, 0x00, 0x00, 0x18,
0x0C, 0x4E, 0x02, 0x00, 0x00, 0x1F, 0x86, 0x4C, 0x7E, 0x00, 0x00, 0x0E, 0xC6, 0xE8, 0xEE, 0x00,
0x00, 0x18, 0x43, 0xF8, 0x82, 0x00, 0x00, 0x10, 0x06, 0x4C, 0x03, 0x00, 0x00, 0x30, 0x0C, 0x46,
0x01, 0x00, 0x00, 0x30, 0x18, 0x46, 0x01, 0x00, 0x00, 0x10, 0x18, 0x43, 0x03, 0x00, 0x00, 0x18,
0x10, 0x43, 0x03, 0x00, 0x00, 0x1C, 0x70, 0x41, 0x86, 0x00, 0x00, 0x0F, 0xE0, 0x40, 0xFE, 0x00,
0x00, 0x09, 0x1E, 0x4F, 0x06, 0x00, 0x00, 0x08, 0x30, 0x43, 0x86, 0x00, 0x00, 0x0C, 0x20, 0x41,
0x86, 0x00, 0x00, 0x06, 0x60, 0x40, 0x8C, 0x00, 0x00, 0x07, 0x60, 0x40, 0xB8, 0x00, 0x00, 0x01,
0xE0, 0x41, 0xF0, 0x00, 0x00, 0x00, 0x38, 0xE3, 0x00, 0x00, 0x00, 0x00, 0x0F, 0xBE, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x1F, 0xCF, 0x82, 0x0C, 0x86, 0x46, 0x1F, 0xEF, 0xC3, 0x0C,
0xC6, 0xEE, 0x1C, 0xEC, 0xC7, 0x0C, 0xE6, 0x7C, 0x1C, 0xED, 0x8D, 0x8C, 0xFE, 0x38, 0x1C, 0xED,
0x8D, 0xCC, 0xDE, 0x38, 0x1D, 0xCD, 0xDF, 0xCC, 0xCE, 0x38, 0x1F, 0x8C, 0xF8, 0xEC, 0xC6, 0x38,
0x1F, 0xEC, 0x08, 0x0C, 0xC2, 0x18, 0x1C, 0xEC, 0x00, 0xC0, 0x00, 0x00, 0x1C, 0xFD, 0xFB, 0xC0,
0x00, 0x00, 0x1C, 0xFC, 0x63, 0x00, 0x00, 0x00, 0x1C, 0xEC, 0x63, 0xC0, 0x00, 0x00, 0x1F, 0xEC,
0x60, 0xC0, 0x00, 0x00, 0x1F, 0xCC, 0x63, 0xC0, 0x00, 0x00, 0x1F, 0x0C, 0x63, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x28, 0x2B, 0x4F, 0x67,
0x42, 0x38, 0x7B, 0xEA, 0x86, 0xB2, 0x28, 0xC7,
};
void draw(void) {
u8g.drawBitmapP( 37, 0, 6, 50, brainy_bitmap); // put bitmap
u8g.setFont(u8g_font_profont11); // select font
u8g.drawStr(0, 15, "T: "); // put string of display at position X, Y
u8g.drawStr(0, 35, "H: ");
u8g.setPrintPos(15, 15); // set position
u8g.print(DHT.temperature, 0); // display temperature from DHT11
u8g.drawStr(30, 15, "c ");
u8g.setPrintPos(15, 35);
u8g.print(DHT.humidity, 0); // display humidity from DHT11
u8g.drawStr(30, 35, "% ");
}
void setup(void) {
analogWrite(backlight_pin, 50); /* Set the Backlight intensity */
}
void loop(void) {
DHT.read11(dht_apin); // Read apin on DHT11
u8g.firstPage();
do {
draw();
} while( u8g.nextPage() );
delay(5000); // Delay of 5sec before accessing DHT11 (min - 2sec)
}
TUTORIAL VIDEO
DOWNLOAD
Copy and paste the above code in the Arduino IDE to program your Arduino.
Used Libraries:
Download LCD Assistant software:
Download the U8GLib library here: https://bintray.com/olikraus/u8glib/Arduino/1.17
Download the DHT library here: https://github.com/RobTillaart/Arduino/tree/master/libraries/DHTlib
Comments